Serverless functions in Go
What are Serverless Functions and where can I use them?
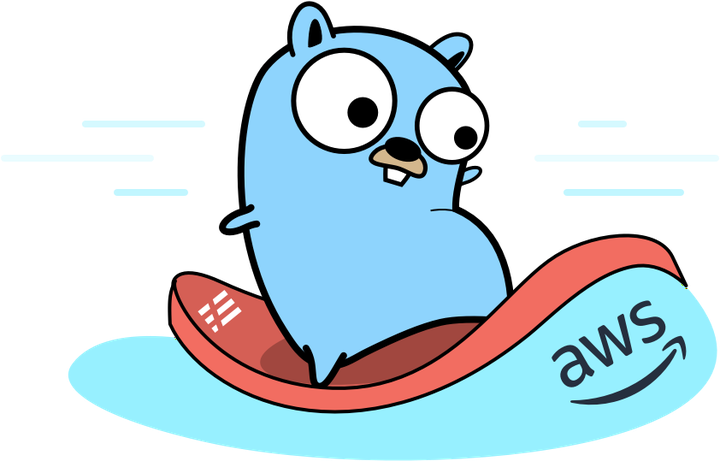
Building a Serverless Function
Maybe you’ve heard about serverless functions (or the term Function as a Service or FaaS) in the past. If not, please read on! You have nothing to lose!
You might hear this term serverless functions or cloud functions more often lately. I’ve heard about these a long time ago but I did lack a good use-case where I could leverage it fully.
Serverless functions
These are functions, that usually execute one simple task or have a single purpose. Imagine serverless functions as something where you expose an API endpoint, that if called, will run your function.
These functions are hosted on a managed infrastructure at a cloud provider. These hosted functions have a high uptime, that means nearly zero downtime in most cases. This makes them quite reliable. The functions are highly scalable and can handle high traffic and demand.
What is even better in all of this, most cloud providers give you a free tier with which you can run your cloud functions for free.
Among other benefits we can also count the costs. At most of the cloud providers you get a pricing that states that you only pay for computation resources when you are using them. What does this mean? In simple terms, if you don’t call your function for a month, you won’t pay a dime for it. You do not need to run a server anymore, configure your own webserver or getting an SSL certificate. Most of the mentioned products is included in the mentioned features.
Platforms
There is a growing range of providers that support Function as a Service. Here is a shortlist but by no means a complete list, I’ve tried to summarize the popular ones, but there are many others out there, you just need to look :).
- AWS Lambda
- Netlify Functions
- Vercel (formerly Zeit)
- Microsoft Azure Functions
- Google Cloud Functions (GCF)
- IBM Cloud Functions
Every one of these has a free tier as I’ve mentioned earlier, due to this it is really simple and easy for anyone to get familiar with the concept and deployment. There would be a really lengthy article if we would like to look at the benefits of each of these providers but let’s keep that for a separate article.
Use-case of serverless
We have to keep mind that serverless architecture is not suitable for every use-case or project. These functions are stateless, so you should build them that way. If you use serverless functions, you are not able to run a database next to it, because there is no server right?
Some providers give you access to cloud databases too, so this can be partly mitigated by this, but it imposes new costs for you.
Let’s build a function
Various cloud providers support various languages for the cloud functions. The most popular one will be likely Javascript, but there is also support for Go, Python, Java, C# and more. So I think developers have a wide variety of support on what language to use and deploy as a function.
I’ve chosen Go for my sample. I love Go, you can build simple, reliable and also efficient software with it. I don’t want to start a war on what language is better or why. I suggest you pick one of the mentioned languages above and deploy the functions. It is really up to you and the platform you are chosing.
Cloud function that parses a webpage
I am building a mobile app for my own personal use, also for a very limited scope of family members that are interested.
I live in a small village, which has an official website where informations are published. This website does not support subscribing to the news (short advertisements, important information), so you need to constantly check the webpage and refresh it if there is anything new.
This is not really ideal for anyone nowadays and a convenient way to display these in a mobile app could be much more useful.
This is where serverless comes into picture. So we have one purpose, parse the page in a consumable format (e.g. JSON) and then output it for our API.
The app is used by a limited number of people, so this means we won’t be flooding the website of the village and still get our data in our mobile app in a more convenient way than before. This was a clear call for a serverless function.
Building the serverless function
As I’ve mentioned I’ve chosen Go for my example because I work with it a lot. I will now show you a simple example on how you can build your own serverless function.
We will use gorilla/mux for routing and ease of use. You can see a sample repo at the end of the article with the results. This can be easily forked and deployed through Netlify.
We will also use the AWS Lambda Go Api Proxy, this proxy allows us to easily define routes and build an API like experience.
Let’s start with our function that will return us the current date with time. Basically time.Now()
. We need a http function handler:
func getCurrentDate(writer http.ResponseWriter, request *http.Request) {
date := time.Now().String()
fmt.Fprintf(writer, date)
}
This is very simple, we get the string format for time.Now
and then using the writer we write the date using the http.ResponseWriter
. Now this is all nice and easy, but we need some simple routing so we have a path to call our function.
For this we will use gorilla/mux
. I would like to get the current date string when I call the path /today
. This makes it really straightforward on what this path does and how to get the current date.
// we need the lambda Adapter, this is where we will pass our routing
// this is basically our proxy
var gorillaLambda *gorillamux.GorillaMuxAdapter
// We will use the builtin init function to setup the router
func init() {
r := mux.NewRouter()
// we define a /today path, allowing only GET method
r.HandleFunc("/today", getCurrentDate).Methods("GET")
gorillaLambda = gorillamux.New(r)
}
I’ve commented the lines, now we have a routing setup. The init()
functions runs before the main function. A package can have multiple init functions, so be careful while using it :)!
Source: https://stackoverflow.com/questions/24790175/when-is-the-init-function-run
But the get back on topic. Now we have our router initialized, our function to return the date ready, we now need a Handler that will handle the incoming requests and pass them to the lambda functions.
func Handler(ctx context.Context, req events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
// If no name is provided in the HTTP request body, throw an error
return gorillaLambda.ProxyWithContext(ctx, req)
}
This will handle the incoming requests and proxy them to the lambda functions, using the router definition we’ve made earlier.
We now need a main()
function, which will combine all of the parts we’ve made earlier into one.
func main() {
lambda.Start(Handler)
}
Now you’re almost ready to deploy your lambda function to Netlify. I will give you a link to my sample repo. This article is not covering the deployment tasks, nor the building process, but basically we need to build our functions, setup a redirect on netlify, to have a simple URL. After all of this, netlify does the rest and deploys your functions to the cloud.
After deployment, you can call the endpoint under a similar url: somerandomdomain.netlify.app/today
and this will out put you something like this:
2020-10-11 20:16:34.831893709 +0000 UTC m=+0.048955809
Sample repo: https://github.com/0x111/serverless-sample
If you have any questions, create an issue or feel free to contact me on my mail. Also please follow me on the socials that you can see below, it would mean a lot to me! Stay tuned for more interesting articles from the tech world!